Building Custom Models with PyTorch Lightning
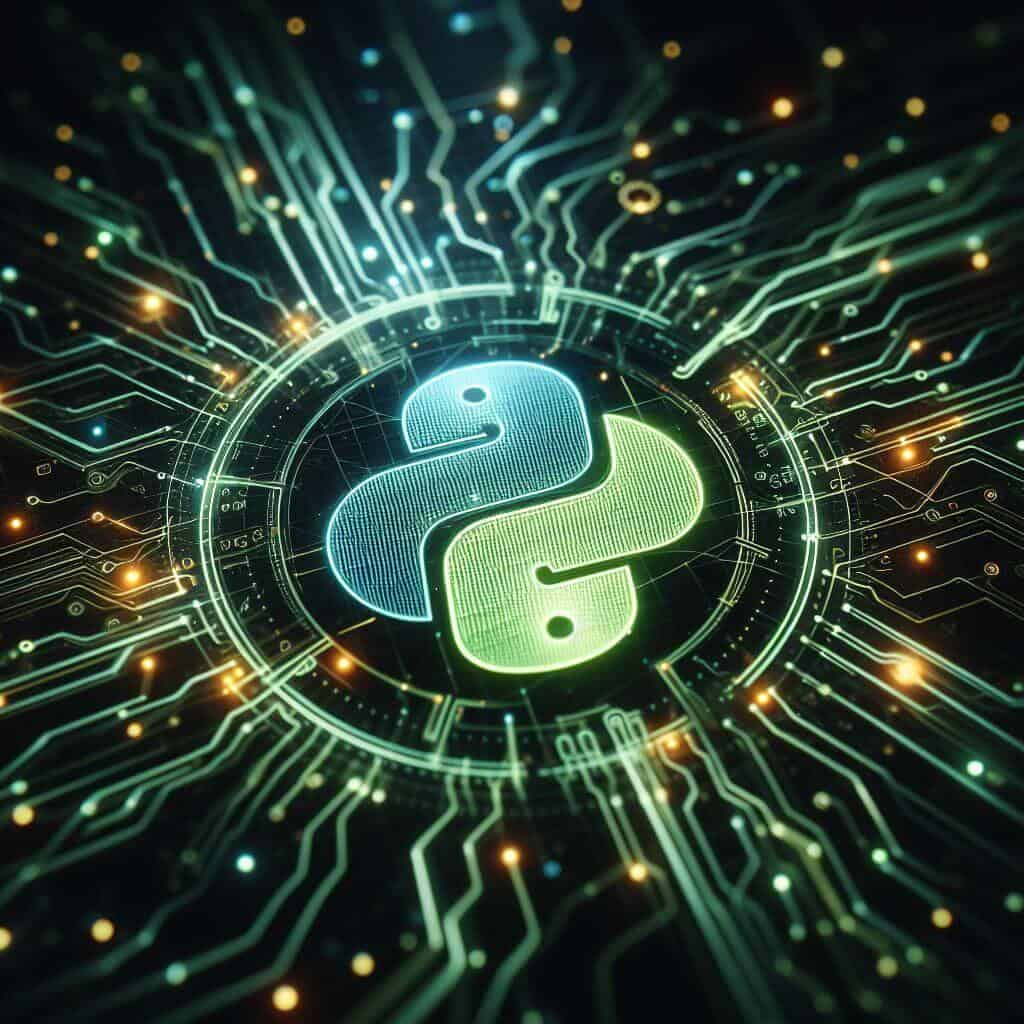
- March 1, 2024
- allix
- AI Education
Building custom models with PyTorch Lightning offers a simplified and efficient approach to deep learning development, allowing developers to focus more on the architecture and logic of their models, rather than the boilerplate code often associated with such tasks.
Getting started with PyTorch Lightning is the first step to making your deep learning projects easier and more efficient. PyTorch Lightning is built on top of PyTorch, one of the most popular deep-learning frameworks available today. Its main purpose is to help you focus on building models instead of getting bogged down in code that is not directly related to the logic or architecture of your model.
To get started, you’ll need to install PyTorch Lightning. This is easy to do with pip, the Python package installer. When you open a command line or terminal, you simply type:
pip install pytorch-lightning
This command will get PyTorch Lightning and install it on your machine if you already have Python and pip set up. It’s a simple process that doesn’t require any special settings or configurations.
Once installed, the path to creating custom models with PyTorch Lightning begins with understanding its core component, the LightningModule. This module acts as an extended version of PyTorch’s own “module”, serving not only to define the model layers but also to encapsulate all the training logic, data flow, and optimization process. It’s designed to keep everything organized and easy to manage, allowing you to write less code and reducing the risk of errors.
The great thing about PyTorch Lightning is that it works with the PyTorch ecosystem, meaning you don’t have to learn a whole new library. Your existing knowledge of PyTorch, such as how tensors work and how neural networks are built, will come in handy. PyTorch Lightning simply adds a structured framework on top, helping to manage and automate many tasks that would otherwise require manual coding.
Creating Your Model
When it comes to building your model with PyTorch Lightning, think of it like drawing a blueprint before building a building. This step is all about planning what your model will look like based on the problem you are trying to solve. For example, if your task is to recognize objects in pictures, you might decide to use layers that are good at capturing images.
Creating your model in PyTorch Lightning involves writing a class that is based on `LightningModule`. This is not too different from how you use `torch.nn.Module` in PyTorch but with the added functionality and structure provided by PyTorch Lightning. In this class, you’ll define the building blocks of your model, such as its layers, and describe how data flows through the model as it runs. This includes everything from how it accepts input to how it makes predictions.
Here’s how to get started:
import pytorch_lightning as pl
imported torch
from the torch imported pp
import torch.nn.functional as F
class CustomModel(pl.LightningModule):
def __init__(self):
super(CustomModel, self).__init__()
# Define the model layers
self.layer1 = nn.Linear(in_features=10, out_features=20)
# You can add more layers if needed
def forward(self, x):
# This is where you define the feed-forward, i.e. how the data is moved through the model
x = self.layer1(x)
return x
In addition to simply describing the architecture of the model, PyTorch Lightning’s `LightningModule` also invites you to include the details of how the model should learn from the data. This includes determining how to calculate training losses and choosing an optimizer that adjusts your model’s parameters (its weights and biases) to reduce this loss over time.
This design step is important because it directly affects the performance of your model. The goal is always to create a model that can make accurate predictions. However, remember that trial and error is often used. It’s okay to go back and tweak the design of your model after you’ve tested it and seen it work.
Training And Evaluating Your Model
Once you’ve set up your model design in PyTorch Lightning, the next step is to train it to make accurate predictions. The idea is to give examples of your model, let it make predictions, see where it goes wrong, and then tweak it a bit to make it better next time. This process is repeated in many examples and many cycles.
Training your model with PyTorch Lightning involves passing data, which is usually broken down into small groups called batches. For each batch, the model tries to predict the outcome, compares its predictions with the actual results, and then corrects itself based on its errors. In technical terms, this involves calculating a “loss” that measures how far off the predictions are, and then using an “optimizer” to make adjustments.
Here’s a simple look at what training settings might include:
from torch.utils.data import DataLoader, TensorDataset
import pytorch_lightning as pl
# Assuming x_data and y_data are your functions and labels
dataset = TensorDataset(x_data, y_data)
dataloader = DataLoader(dataset, batch_size=32)
# Initialize your beautifully designed model
model = CustomModel()
# The PyTorch Lightning trainer handles the training cycle
trainer = pl.Trainer(max_epochs=10)
# Start learning
trainer.fit(model, dataloader)
In this setup, you tell Trainer how many times to go through the entire dataset (epochs) and it will take care of the rest, including starting the training loop, grouping the data, and calling your `forward`, `training_step`, and `configure_optimizers` methods at the right time. That’s a lot less coding for you and less room for error.
But learning is only half the battle. To know how good your model is, you need to test it. This is called evaluation, and it’s like a game day where you see how well your team (or model) is doing. For a machine learning model, this usually means testing how it performs on data it hasn’t seen before, often called “validation” or “test” data. This is an important step because it can tell you whether your model has learned general rules about your data or just memorized the training examples.
PyTorch Lightning makes evaluating your model easy. You can set up a separate DataLoader for your test data and use the `trainer.test` method:
test_dataset = TensorDataset(x_test_data, y_test_data)
test_dataloader = DataLoader(test_dataset, batch_size=32)
# Check the model
trainer.test(test_dataloader)
This step gives you a clear picture of how your model performs on new, unknown data, which is the ultimate test of its performance.
Advanced Customization And Flexibility
As you dive deeper into modeling with PyTorch Lightning, you’ll find that it doesn’t just simplify the basics—it also includes a lot for those who want to do more complex things. Imagine you’ve been driving a car with an automatic transmission, and suddenly you’re given a car that can shift into manual mode, giving you more control when you want it. PyTorch Lightning is similar in that it provides advanced features and flexibility for those times when you need to fine-tune your model or experiment with something new.
One such area you might want to explore is adjusting how your model learns over time, such as changing the learning rate as it trains. In many projects, starting with a higher learning rate and then decreasing it may allow your model to learn faster at first and fine-tune towards the end. PyTorch Lightning lets you do this seamlessly with scheduling features. Here’s how you can adjust the learning rate over time in your `LightningModule`:
def configure_optimizers(self):
optimizer = torch.optim.Adam(self.parameters(), lr=1e-3)
lr_scheduler = torch.optim.lr_scheduler.StepLR(optimizer, step_size=4, gamma=0.1)
return [optimizer], [lr_scheduler]
This piece of code tells PyTorch Lightning to use an optimizer that adjusts the learning rate after every 4 steps, reducing it to 10% of the previous value each time. This is an easy way to greatly influence how well and how quickly your model learns.
But that’s not all. PyTorch Lightning’s ability to let you customize goes much further. Let’s say you’re working on a project that requires a lot of computing power, and you have access to multiple GPUs or even a cluster of machines. PyTorch Lightning makes it easy to extend your training with just a few changes to the “trainer” settings. You don’t need to rewrite your model or dive into the intricate details of distributed computing. PyTorch Lightning will handle the hard parts for you.
Finally, another great feature of PyTorch Lightning is the built-in support for logging and monitoring your training progress. Whether you’re watching your losses decrease over time or want to gain a deeper understanding of how your model’s performance changes with each epoch, PyTorch Lightning easily integrates with tools like TensorBoard. This means you can visualize your model’s training progress, helping you make informed decisions about how to adjust the training process for better results.
Categories
- AI Education (33)
- AI in Business (58)
- AI Projects (80)
- Research (53)
Other posts
- Meta Introduced Llama 3 LLM
- Caffe – A Deep Learning Powerhouse
- The Study Reveals The Potential Of Artificial Intelligence To Take Over Key Management Functions In Scientific Research
- eBay Introduces New AI-Driven ‘Shop the Look’ Functionality to Its iOS Application
- Brave Releases Ai Assistant for iPhone and iPad Users
- Introduction to MXNet
- The Quiet-STAR Algorithm, Which Allows Chatbots To Think About Answers Before Responding
- Orchard’s Vision System Transforms Agricultural Machinery Into Ai-Powered Data Collection Tools
- Deepmind Introduces Genie – An Artificial Intelligence That Creates 2d Game Environments From A Single Image
- Microsoft Cognitive Toolkit (CNTK)
Newsletter
Get regular updates on data science, artificial intelligence, machine