Theano Overview and Guide
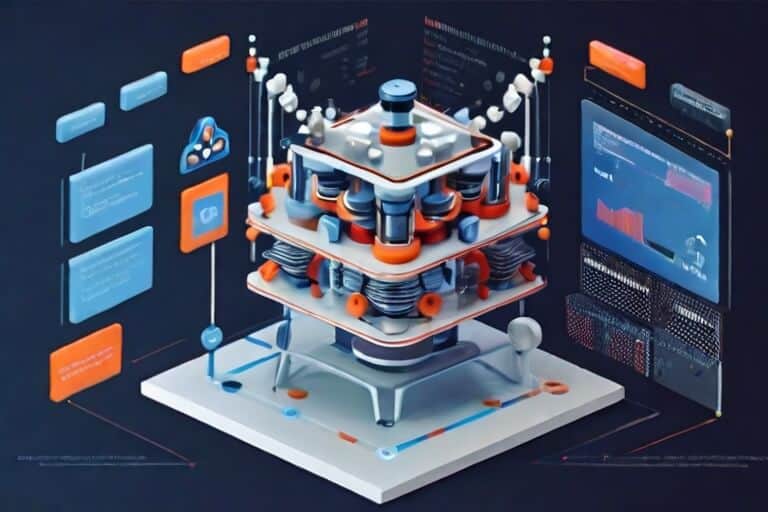
- January 26, 2024
- allix
- AI Education
In the field of deep learning, Theano has become a very influential and powerful tool among data scientists and machine learning enthusiasts. At its core, Theano is a computational framework for writing and optimizing mathematical expressions involving multidimensional arrays, a staple in the world of artificial neural networks. It is designed for computationally intensive tasks that are a hallmark of deep learning, making it a key resource for those developing complex AI applications.
Theano’s architecture allows for seamless integration with other Python libraries, such as NumPy, which is known for its efficient processing of numerical data. This integration means that Theano can manipulate NumPy arrays with extreme agility, using the convenience of NumPy and Theano’s own optimizations for high-performance computing. Given the large-scale nature of deep learning tasks, where datasets and model parameters can be huge, the synergy between Theano and NumPy is particularly beneficial.
Dynamic C code generation, one of Theano’s most important features, further cements its status as a powerhouse. It dynamically generates optimized C code for functions on the fly, carefully managing low-level details such as memory allocation and loop unwrapping. This feature allows Theano to approach, and sometimes even exceed, the speed of handwritten C code while maintaining the readability and lightness of Python code. Consequently, developers spend less time worrying about performance bottlenecks and more time focusing on actually designing and improving deep learning models.
Theano Setup and Basics
Starting your deep learning with Theano starts with setting up the right development environment. This setup should include not only Theano but also the supporting libraries and dependencies that allow it to perform at its peak. Installation is initiated by a simple pip command, which is Python’s package installation mechanism. To meet the specific needs of Theano, additional components such as NumPy—the core for numerical computation in Python—should be integrated along with a BLAS (Basic Linear Algebra Subprograms) implementation to improve computational efficiency. They are the basis for the high-level scientific computing that Theano uses to perform complex matrix and vector operations efficiently.
After installation, it’s a good idea to run a few checks to make sure Theano is configured correctly. Verifying the installation involves running the test scripts provided in the Theano package. This step is vital, as it helps you spot any potential misconfigurations or compatibility issues early that could hinder your work. Additionally, familiarization with the installed version of Theano is important for compatibility with existing guides, scripts, and community resources.
After installation and verification is complete, the next step is to learn the basics of Theano’s computational model. At the core of Theano’s computing architecture is the concept of symbolic computing. Unlike traditional imperative programming, where statements are executed sequentially, symbolic programming involves constructing a representation of the computation itself, not necessarily its immediate execution. This abstraction is represented as a computational graph in Theano, which details the mathematical relationships between variables without assigning them explicit values.
In this graphing paradigm, symbolic variables are placeholders for the data to be entered into the graph. These variables are strongly typed, indicating their data type and dimension – scalar, vector, matrix, or tensor. Defining these variables is like declaring the type and shape of the data you plan to work with without specifying their actual values.
Creating and manipulating symbolic variables in Theano is simple yet powerful. To demonstrate, by declaring symbolic floating-point scalars, you are defining abstract entities that will be part of the algebraic expressions in your deep-learning model. When it’s time to do the actual calculations, these symbolic expressions are compiled into Theano functions. These functions are efficient because they are translated into low-level code, bypassing Python’s interpretation overhead. Thus, compiling in Theano is not only about executing a defined graph but also about turning it into highly optimized code that is ready to run efficiently on hardware, whether it’s CPU or GPU.
Building deep learning models with Theano
A common starting point for model creation is the implementation of a logistic regression classifier, a relatively simple but fundamental structure in the neural network universe. This involves defining variable parameters, such as weights and biases, and defining mathematical relationships for predictions, such as the softmax function for categorical outputs. You will then use the loss function to quantify the discrepancy between the model predictions and the actual target values. A popular choice for multiclass classification problems is the cross-entropy loss, which captures the difference between the predicted probability distribution and the true distribution.
To illustrate these concepts with a concrete example, let’s look at the procedure for setting up a logistic regression classifier in Theano. You could start by introducing shared variables to hold model parameters—these shared variables are Theano’s mechanism for saving state while functions are executed. Shared variables such as the weight matrix “W” and the bias vector “b” are then included in symbolic expressions that define the model’s operation.
W = theano.shared(
value=np.zeros((n_in, n_out), dtype=theano.config.floatX),
name=’W’,
borrow=True
)
b = theano.shared(
value=np.zeros((n_out,), dtype=theano.config.floatX),
name=’b’,
borrow=True
)
Once everything is ready, the next step is to create symbolic expressions for model predictions, calculate losses, and update parameters during training. An optimization algorithm is required for training—stochastic gradient descent (SGD) is a classic choice due to its simplicity and efficiency. In SGD, the parameters are iteratively adjusted in the opposite direction of the gradient, scaled by the learning rate.
This is where Theano feature compilation comes into play, where features are compiled to handle updates received from SGD during training iterations. These functions encapsulate everything from direct pass calculations to gradient calculations and update steps optimized for execution speed.
Optimization and Debugging
The benefits of Theano’s optimization capabilities are that they are easily accessible, yet extremely complex. Optimization flags can be set either globally in the Theano configuration file or locally by specifying options when compiling functions. These flags control various aspects of Theano’s behavior, such as the aggressiveness of computational graph optimization, the choice between CPU and GPU execution, and the precision of math operations. By adjusting these settings, developers can significantly change the performance characteristics of their models.
For example, the choice of optimizer can significantly affect both the execution time and memory consumption of the model. The default Theano optimizer ‘fast_run’ provides a balance between speed and stability, but users can also choose ‘fast_compile’ for faster compile times during development or ‘merge’ to aggressively minimize the number of graph nodes, which can be especially useful for very large models.
The profiling tools in Theano represent an additional level of introspection into the computing process. When compiling with profiling enabled, the profiling report shows where time and memory are being consumed in models and operations. This breakdown enables developers to pinpoint bottlenecks or inefficiencies that might otherwise remain hidden, such as redundant computations or memory access patterns that prevent parallel execution.
Enabling profiling might look like this:
f = theano.function(inputs=[x, y], outputs=z, mode=theano.compile.ProfileMode())
After the `f` function is executed, Theano will collect and provide timing and memory usage information for each operation in the calculation graph. Understanding this result can be transformative, guiding model optimization strategies and shedding light on unexpected behavior.
Coupled with its optimization prowess, Theano offers robust debugging tools, an often overlooked but important feature set. Debugging in Theano is made easy with expressive error messages and an advanced “NanGuardMode” debugging mode that monitors computations and warns users about numeric instabilities such as NaN (not a number) or Infs (infinity) when they occur.
This support for defensive programming is complemented by Theano’s visualization capabilities. Using `theano.printing.pydotprint`, one can generate graphical representations of a computational graph. This visualization simplifies the understanding and debugging of complex models by presenting data and computational flow in a convenient and visual format.
Categories
- AI Education (33)
- AI in Business (58)
- AI Projects (80)
- Research (53)
Other posts
- Meta Introduced Llama 3 LLM
- Caffe – A Deep Learning Powerhouse
- The Study Reveals The Potential Of Artificial Intelligence To Take Over Key Management Functions In Scientific Research
- eBay Introduces New AI-Driven ‘Shop the Look’ Functionality to Its iOS Application
- Brave Releases Ai Assistant for iPhone and iPad Users
- Introduction to MXNet
- The Quiet-STAR Algorithm, Which Allows Chatbots To Think About Answers Before Responding
- Orchard’s Vision System Transforms Agricultural Machinery Into Ai-Powered Data Collection Tools
- Deepmind Introduces Genie – An Artificial Intelligence That Creates 2d Game Environments From A Single Image
- Microsoft Cognitive Toolkit (CNTK)
Newsletter
Get regular updates on data science, artificial intelligence, machine